Several days ago, Monday April 4th 2016, was a very important day for me as well as for my company. I reached a huge milestone, even though it may not seem like a big think when you look at it more closely. And you would be absolutely right, but still: I couldn’t be more excited about it than I already am.
So what’s all this about, you may ask? I’ll tell you: last Monday, my ixudra/curl package made it to the 10.000 download mark! Let me say that again: 10.000 lifetime downloads! That’s insane! Now to fully understand what I’m trying to tell you here, you need to have some understanding of PHP web development in general and PHP packages in particular. Odds are, if you’re reading this blog post, you already have a firm handle on this, in which case feel free to skip the next 3 paragraphs and dive right into the good stuff. If not, I will quickly lay it out for you so you at least know more or less what I’m talking about.
In software development (not just PHP), developers all around the world are constantly working on similar projects, performing similar tasks and facing similar problems. This is very normal due to the very nature of software development. This causes a lot of duplicate code, since lots of people try to solve these problems in a (more of less) identical way. This is not very productive and doesn’t help anyone in the long run.
In order to prevent this from happening, developers all over the world have started working together on common problems and distributing their solutions via independent and reusable modules. These modules can tackle a wide variety of problems, such as connecting to Facebook, managing appointments across different time zones, collecting traffic information, you name it. The possibilities are limitless. And in PHP, these modules are called packages.
This technique was a huge step forward for software development, but it gets even better. Rather than keeping their modules to themselves, developers have created a distribution platform to share their modules with other developers from all around the world, (usually) free of charge. This was a huge game changer: it literally gives a 14-year old kid with a dial-up modem in his parent’s basement in India the ability to change the world and help thousands of people, just by providing an elegant solution to a common developer problem. In PHP, this distribution platform is called composer. Now, back to the original story.
Ever since I started working in PHP, I’ve been fascinated by the concept of packages and how they can make your life as a developer so much easier. The idea of creating packages myself immediately spoke to me, but I still had a lot to learn before I could actually get started on it. It also seemed like I didn’t have anything to contribute to the community, which is a common misconception that people have, especially software developers as they tend to be more introverted.
It wasn't until a year later that I actually got my head out of my ass and created my first package. And so, ixudra/curl was born. At the time, I was working for Cegeka and I was doing a rewrite of an existing project in the Laravel 4 framework (which was the latest version at the time). The original code was very poorly written and it took a lot of hard work not only to make it work, but also me make the code readable, understandable and elegant for other developers that were eventually going to pick up where I left off.
I pulled in several packages to make this all work out, but some aspects of the code I just couldn’t get them to look (and handle) the way I wanted them to. The code was functional, don’t get me wrong: it did exactly what it was supposed to do, but it still felt wrong in more ways than one.
```
protected function doRequest($getParameters = '', $postParameters = false, $processType = 'json')
{
if( is_array($getParameters) && count($getParameters) != 0 ) {
$getParameters = '?'.http_build_query($getParameters);
} else {
$getParameters = '';
}
$curl = curl_init( $this->getEndpoint() .'/'. $this->getAction() . $getParameters );
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
if( $postParameters !== false ) {
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($postParameters));
curl_setopt($curl, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json'
));
}
$curlResponse = curl_exec($curl);
curl_close($curl);
if( $processType == 'json' ) {
return json_decode($curlResponse);
}
return $curlResponse;
}
The above code sample shows the original code as I received it from the previous development team. As for what is happening here, it’s very simple: we require some information that is stored in another server. In order to collect that information, we need to send a request to the server that stores it, recover the data it sends back to us and transfer it to the user. In PHP, this is done using the cURL protocol.
There is no need to understand exactly what is going on here. What is important, is the blatantly obvious: it’s messy (to put it mildly). In order to make it more intuitive, I decided to take it upon myself and create a package that would take care of all of the ugliness in front of me and make the structure a little bit more fluent, more elegant. And this is what I came up with:
```
protected function doRequest($getParameters = '', $postParameters = false, $isJson = true)
{
$url = $this->getEndpoint() .'/'. $this->getAction();
if( $postParameters !== false ) {
return Curl::post($url, $getParameters, $postParameters, $isJson);
}
return Curl::get($url, $getParameters, $isJson);
}
Even to the untrained eye, the differences should be immediately obvious. The code changes may seem significant, but in truth, all I did was move the more complex and unreadable parts of the code to a different location and provided an easy access point via a clear interface. In short: I improved readability without sacrificing functionality and therefor increased the quality of the code. Mission accomplished.
Over a year went by without anyone ever noticing the package even existed. Initially, I was the only one that used on occasion, which made perfect sense: I made it specifically for my own purposes. It might have been useful to someone else, but that was more by coincidence than by design. There was the occasional download here and there, but suffice it to say that nobody knew and nobody cared.
Things started moving after I published several posts on the official Laravel forum and on the Laracasts forum asking for feedback on my packages, one of which was the curl package. I got a lot of great feedback, some negative, but I did notice a small increase in the number of downloads per week. Soon, I reached my first 1.000 downloads, and I was as happy as a kid at Christmas.
The big change didn't come until October last year. I hadn't done any work on the package since I originally made it, except for some minor bug fixes and other small tweaks. In order to further increase the usability and the ease of use of the package, I decided to change things up to use a fluent interface. The inspiration came from the Fluent query builder that Taylor Otwell created for the Laravel framework.
```
// Send a GET request to: http://www.foo.com/bar?foz=baz using JSON
$response = Curl::to('http://www.foo.com/bar')
->withData( array( 'foz' => 'baz' ) )
->asJson()
->get();
// Send a POST request to: http://www.foo.com/bar with arguments 'foz' = 'baz' using JSON and return as associative array
$response = Curl::to('http://www.foo.com/bar')
->withData( array( 'foz' => 'baz' ) )
->asJson( true )
->post();
These changes proved to be one of the best things I've ever done as a software developer. Not only did it allow for more flexibility on the part of the developers, it also increased readability and made it possible to completely configure the curl request in a way that was very familiar to Laravel developers.
After the changes, the amount of downloads per day started to increase dramatically, even though I never did any additional promotion. Soon, I started receiving emails and tweets about the package from total strangers, thanking me for my contributions.
<div style="text-align: center;"> [ 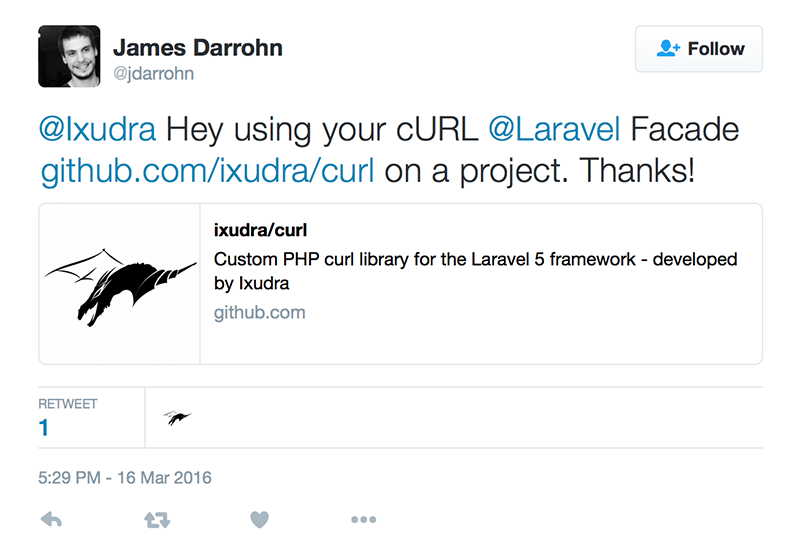 ](https://twitter.com/jdarrohn/status/710261862558990336) </div> The package was also mentioned in the [PHPWeekly newsletter](http://www.phpweekly.com/archive/2016-01-14.html) under the header "Interesting tools, libraries and projects". I wasn't contacted about this, I don't even know how this selection is made. I actually found out by coincidence when I was checking the statistics on the [package Github page](http://github.com/ixudra/curl). I have no idea what impact this has had on the popularity of the package, but certainly it made me a very happy developer.
Which brings us to today. As of this week, the average number of downloads has increased to over 2.000 per month, with a total 10.000 downloads since the package was originally added to composer.
Now what does the number actually mean? It means that my code has been downloaded and used by somewhere between 5.000 and 8.000 individual people all across the world in multiple projects, solving a wide range of problems. This has gone far beyond my wildest dreams and it's an incredible feeling to know that I've been able to help to many people with just a couple of lines of code... And I want more!
For me, this has been an amazing ride and I hope I can keep it going for a good long while. And not just for this package: I hope this is the first in a long line of small, medium or large contributions to the PHP community. I'm aware that I still have a long way to go and there is still a lot to learn, but I'm very eager to get started after having achieved this milestone.
But it's more than just a milestone for me. This has actually been the first time in my life I've been able to make any kind of impact in the life of other people outside of my immediate environment. And that doesn't mean that I've never helped other people, through my work or otherwise, or that my package is so much better everything else on the market. In fact, there are other packages out there such as Guzzle that are far more extensive and complete But this one is special to me because it's my own. In a certain way, I even consider this my first successful venture in the startup world since I was able to launch my own "product" into an existing ecosystem and in effect gathered over 10.000 happy customers within the 2 years. I don't know about you but I'd consider that a success.
So what's next? I fully intend to continue working on my curl package and create many more that will follow it. There is no guarantee that my success will continue or that I will ever be able to repeat it, but the only way to change the status quo is by going out there and making something happen, so that's exactly what I'm going to do. My ultimate goal is to be able to create something as beautiful and significant as Taylor Otwell's [Laravel Spark](https://mattstauffer.co/blog/introducing-laravel-spark-a-deep-dive), which is about to released in the next couple of weeks. I might even be able to transform this into business venture instead of just making open source contributions, who knows? To quote Robert Frost:
> The woods are lovely, dark and deep,
> But I have promises to keep,
> And miles to go before I sleep,
> And miles to go before I sleep.
0 Comments on this post yet